Today I am going to tackle a very simple problem of QR code decoding with Next.js 14 API routes example program. We will create a very simple API route for QR code decoding. We are using the jsQR library. As with the new app router support in the Next.js, now the API routes are written with the route.js
files inside any folder of your choice. Which means now any route could serve it’s own API. You can also write server functions which we explain in one of our previous posts. But we are not going to cover that part in this. Our main goal is to use ESP32-CAM to send an image to this API route and that API route will return with the decoding string back to ESP32-CAM.
In today’s post we will stick to the Next.js part of the project so we don’t get distracted. First of all we have to create a new next.js application. Which could be done with simple command like follows
npx create-next-app@latest
Code language: CSS (css)
Which will result in bunch of questions in the command prompt like this image showing the results.
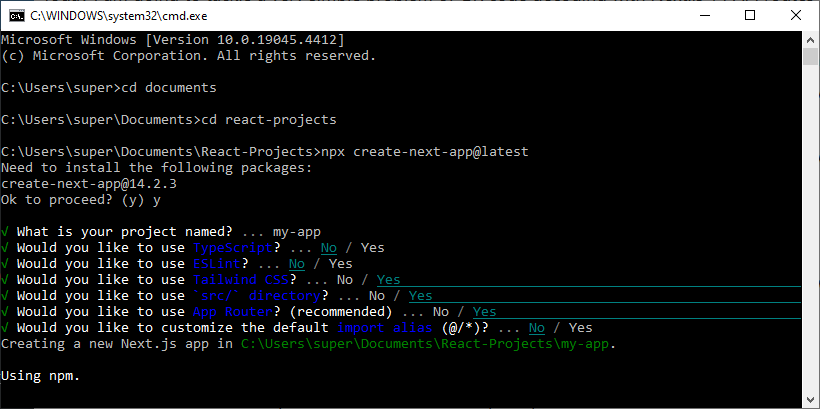
Once done with that, open the folder with the VS Code.
Now create a new api
directory under the src \app
folder. Inside that api folder, create a new file name it route.js
. Because we disabled the typescript support while creating the new project, so we are using the javascript only.
Inside that route.js
file create a simple hello world route with GET method like this.
export async function GET(req, res) {
const name = req.nextUrl.searchParams.get("name") || "World";
return NextResponse.json({ message: `Hello, ${name}!` });
}
Code language: JavaScript (javascript)
This will serve a simple hello world api end point which you can access with like this.

Accessing Base64 Image
Now we have to accep the base64 image in our API and have to convert that back into the image data. There are bunch of libraries and methods to accomplish this but we are going to be as simple as possible and the approach we are using is described in this jsQR issue page.
export async function POST(req) {
const data = await req.json();
const base64Image = data.img;
console.log(base64Image)
}
Code language: JavaScript (javascript)
This is a simple POST method which get the image data provided with the img field as json input to the API end point.
Decode Base64 QR Code Image
Now that we have a base64 image we can try to decode the image with the jsQR library. first we have to import that library then pass an image data to the instance and it will return a bunch of output. We can simply decode that output and get our requried data. This is how we updated our POST route above.
export async function POST(req) {
const data = await req.json();
const base64Image = data.img;
console.log(base64Image)
const imageBuffer = Buffer.from(base64Image, 'base64');
if (imageBuffer[0] === 0x89 && imageBuffer[1] === 0x50) {
console.log('png')
} else if (imageBuffer[0] === 0xFF && imageBuffer[1] === 0xD8) {
console.log('jpeg')
} else {
throw new Error('Unsupported image format');
}
const qrArray = getImageDataFromBuffer(imageBuffer);
const code = jsQR(qrArray.data, qrArray.width, qrArray.height);
return NextResponse.json({ message: code.data });
}
Code language: JavaScript (javascript)
Test API with PostMan
Now we are ready to test our API end point with the postman. We simply pass the base64 encoded as raw input to the API end point. Here is the sample input which we given to the API for our QR code image. We used this simple image to base64 online encoder to generate our base64 encoding. To view and verify that our base64 encoding is correctly working we can use a base64 Image viewer freely available online to validate our base64 encoding. Here is the sample input we provided for the test case.
{
"img":"iVBORw0KGgoAAAANSUhEUgAAASwAAAEsCAYAAAB5fY51AAAABGdBTUEAALGPC/xhBQAAACBjSFJNAAB6JgAAgIQAAPoAAACA6AAAdTAAAOpgAAA6mAAAF3CculE8AAAABmJLR0QAAAAAAAD5Q7t/AAAACXBIWXMAAABgAAAAYADwa0LPAAAF9klEQVR42u3dwW3zRhhFUSv4e5D6r05QE8om2QRZUPBg+F3qnAJk0hYuZvEwvr3f7/cPQMBfZz8AwFGCBWQIFpAhWECGYAEZggVkCBaQIVhAhmABGYIFZAgWkCFYQIZgARmCBWQIFpAhWECGYAEZggVkCBaQIVhAhmABGYIFZAgWkCFYQIZgARmCBWQIFpAhWECGYAEZggVkCBaQIVhAhmABGYIFZAgWkCFYQMafsx/g/9zv95/X63X2Y5zi/X4v+Zzb7bbtZ12V7+E8TlhAhmABGYIFZAgWkCFYQIZgARmCBWQIFpAxcjh6xPP5/Lnf72c/xkdWDRGPjEJXWfWzpg4Rf+ubv4dncMICMgQLyBAsIEOwgAzBAjIEC8gQLCBDsICM7HD0iJ0Dy53DyCM/68i7F0ehxZtUr/o9PIMTFpAhWECGYAEZggVkCBaQIVhAhmABGYIFZFx6OHpVOwefO0ePqxTHpRzjhAVkCBaQIVhAhmABGYIFZAgWkCFYQIZgARmGo0GrRo+rBpbTbi41Cr0uJywgQ7CADMECMgQLyBAsIEOwgAzBAjIEC8i49HD0qgPCaTdqrhqXrhqgFn8/HOOEBWQIFpAhWECGYAEZggVkCBaQIVhAhmABGdnh6OPxOPsR+MfOm0t33oB6hO/hXk5YQIZgARmCBWQIFpAhWECGYAEZggVkCBaQcXu7DpFfmvav6rkuJywgQ7CADMECMgQLyBAsIEOwgAzBAjIEC8gYeePozhsjV5l2E+bO91r1OTsHqMVbUnd+zlROWECGYAEZggVkCBaQIVhAhmABGYIFZAgWkDHyxtFvHlge4YbP39v5HZv2ey6PS52wgAzBAjIEC8gQLCBDsIAMwQIyBAvIECwgY+SNo9Nu71w1opt2o+a0Z975PDsVn3kqJywgQ7CADMECMgQLyBAsIEOwgAzBAjIEC8gYeePooQcP35r42/eaZucodNrfdNq/mC/+Dj/hhAVkCBaQIVhAhmABGYIFZAgWkCFYQIZgARkjbxy9qp2jvp1DxFWKA8vimHPa83zCCQvIECwgQ7CADMECMgQLyBAsIEOwgAzBAjJGDkenjQNXKY5Cp41Ldyq+19X/Xk5YQIZgARmCBWQIFpAhWECGYAEZggVkCBaQMXI4usrOEd0333J59bHijnff+T0sc8ICMgQLyBAsIEOwgAzBAjIEC8gQLCBDsICMSw9Hj5h24+i0W1KPKI4e3f7a5IQFZAgWkCFYQIZgARmCBWQIFpAhWECGYAEZXz8c3TnULI5CV/nm0eOq9zIudcICQgQLyBAsIEOwgAzBAjIEC8gQLCBDsICM2/uqS8ULK97w+c3vPm0wPO15PuGEBWQIFpAhWECGYAEZggVkCBaQIVhAhmABGSNvHL3f7z+v1+vsxzjFqsHezltSp73XtBs+V/0snLCAEMECMgQLyBAsIEOwgAzBAjIEC8gQLCBj5HD0iOfz+XO/389+jI+sGsROuw3yquPJnb/nVYPYad+N1ZywgAzBAjIEC8gQLCBDsIAMwQIyBAvIECwgIzscPWLnEHHavxqf9syrnmfnbaI7GYUe44QFZAgWkCFYQIZgARmCBWQIFpAhWECGYAEZlx6O8ns7x5w7n+eqN3xedVT8LycsIEOwgAzBAjIEC8gQLCBDsIAMwQIyBAvIMBy9qJ3DyOK4dOfnHLFz8DntttVPOGEBGYIFZAgWkCFYQIZgARmCBWQIFpAhWEDGpYejU29NvJriLZfThpqr3r14S+onnLCADMECMgQLyBAsIEOwgAzBAjIEC8gQLCAjOxx9PB5nPwIfmDaw3DmenDbULI9LnbCADMECMgQLyBAsIEOwgAzBAjIEC8gQLCDj9p66EAP4DycsIEOwgAzBAjIEC8gQLCBDsIAMwQIyBAvIECwgQ7CADMECMgQLyBAsIEOwgAzBAjIEC8gQLCBDsIAMwQIyBAvIECwgQ7CADMECMgQLyBAsIEOwgAzBAjIEC8gQLCBDsIAMwQIyBAvIECwgQ7CADMECMgQLyBAsIONvJaiVLry8LTEAAAAldEVYdGRhdGU6Y3JlYXRlADIwMjQtMDUtMjNUMDk6NDM6NTYrMDA6MDBkCKVKAAAAJXRFWHRkYXRlOm1vZGlmeQAyMDI0LTA1LTIzVDA5OjQzOjU2KzAwOjAwFVUd9gAAAABJRU5ErkJggg=="
}
Code language: JSON / JSON with Comments (json)
the output looks like this in the postman software.
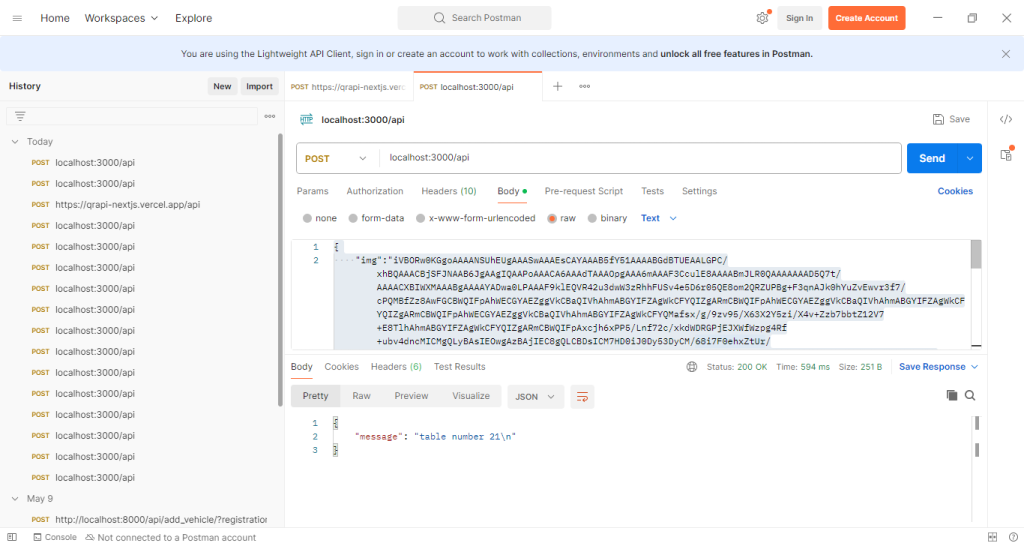